About TwitDown
TwitDown was developed to streamline the process of downloading Twitter videos.
At its core, it's an intuitive interface built on top of a sophisticated video parsing API (to avoid abuse, the API is not explicitly documented in the source code). Users simply input a tweet URL to receive the video download link.
To optimize API utilization and enhance performance, parsed video URLs are cached in our database. Subsequent requests for the same video are served directly from this cache, significantly reducing response times.
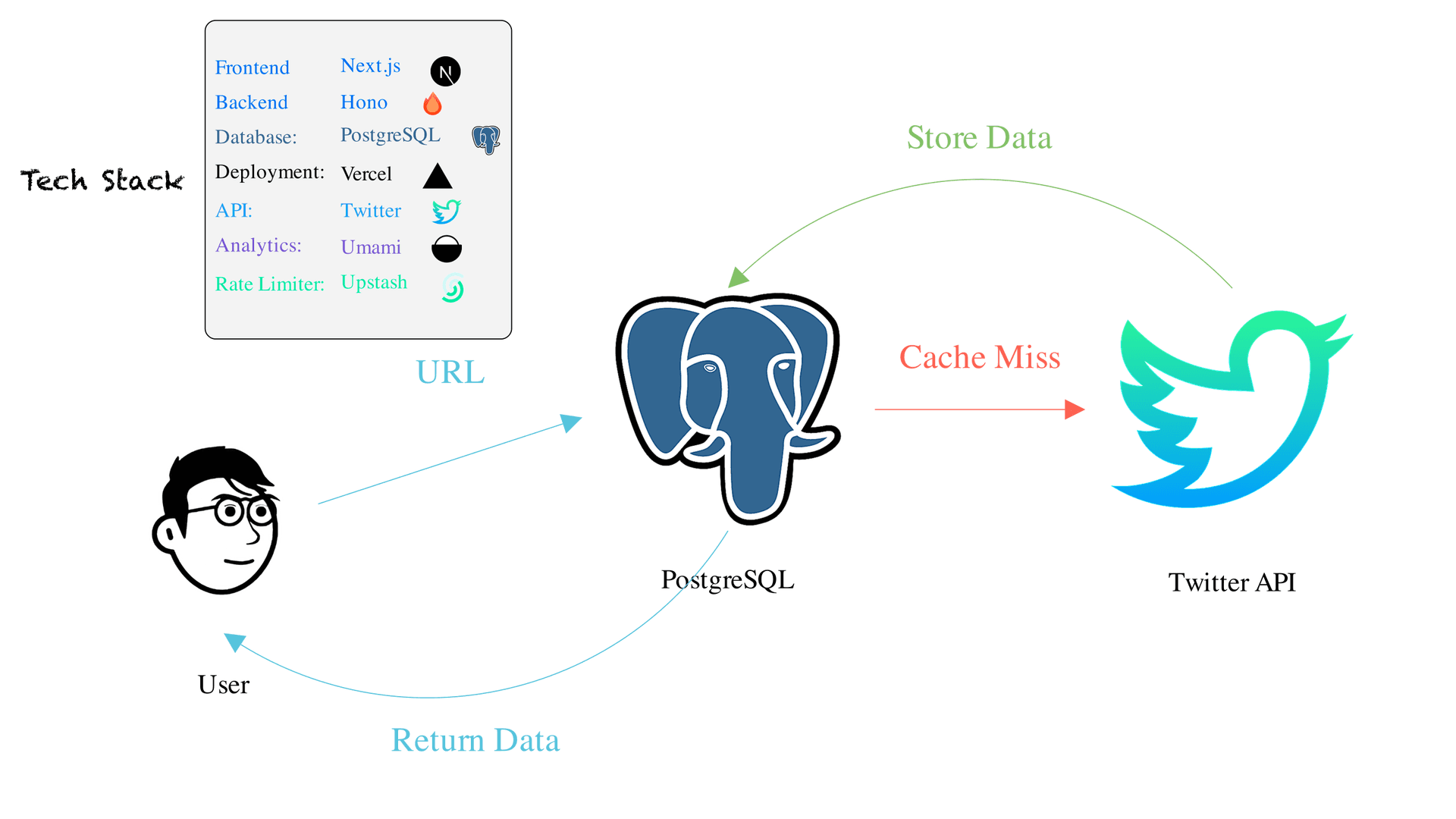
Technology Stack
- Frontend:Next.js (React-based framework)
- Backend:Hono (Lightweight web framework)
- Database:PostgreSQL (Relational database)
- Deployment:Vercel (Cloud platform for static and serverless deployment)
- Video parse API:Twitter API
- Analytics:Umami (Privacy-focused analytics)
- Rate limiter:Upstash (Serverless Redis service)
Why Hono?
The project initially utilized Next.js API routes but transitioned to Hono due to its simplicity and elegance:
- > Familiar paradigm for FastAPI developers - enabling a seamless transition
- > Built-in middleware configuration - offering out-of-the-box functionality
- > Intuitive and clean routing system
- > Simplified implementation of authentication, rate limiting, and other middleware functions
For instance, implementing authentication for /api/v1/download
is remarkably straightforward with Hono. Here's an illustrative example:
import { Hono } from "hono";
import { handle } from "hono/vercel";
import { setupMiddleware } from "../middleware";
import { ApiService } from "../services/api-service";
const app = new Hono().basePath("/api");
// Middleware configuration
setupMiddleware(app);
// Public endpoints
app.get("/hello", (c) => c.json({ message: "Hello, World!" }));
// Protected endpoints
app.get("/analytics", ApiService.handleAnalytics);
app.post("/twitter/parse", ApiService.handleTwitterParse);
export const GET = handle(app);
export const POST = handle(app);
In contrast, Next.js API routes require more extensive setup for middleware:
- > Necessity for a separate middleware.ts file
- > Custom middleware implementation requirements
- > Additional configuration steps in middleware.ts
Next.js Middleware Configuration
import { NextResponse } from "next/server";
import type { NextRequest } from "next/server";
import { checkAuth } from "@/lib/middleware/auth";
import { checkRateLimit } from "@/lib/middleware/rate-limit";
export async function middleware(request: NextRequest) {
if (request.nextUrl.pathname.startsWith("/api/public")) {
return NextResponse.next();
}
// 1. Authentication verification
const authError = await checkAuth(request);
if (authError) return authError;
// 2. Rate limit check for API routes
if (request.nextUrl.pathname.startsWith("/api")) {
const rateLimitError = await checkRateLimit(request);
if (rateLimitError) return rateLimitError;
}
return NextResponse.next();
}
export const config = {
matcher: "/api/:path*",
};
This comparison is not to suggest that Hono is inherently superior to Next.js; rather, its design philosophy aligns more closely with the project's requirements, offering enhanced code readability and maintainability.
The TwitDown project is open-source and available on GitHub ultrasev/twitdown
Search Engine Optimization Strategy
Beyond creating a functional tool for video downloads, this project serves as a practical testbed for implementing advanced SEO techniques.
While developing a website is relatively straightforward, achieving high search engine rankings requires meticulous implementation of SEO techniques.
Leveraging AI-assisted tools like Cursor, numerous optimizations were implemented, including metadata refinement, robots.txt configuration, and sitemap.xml generation. Post-launch analysis using AITDK revealed promising results, confirming proper implementation of titles, descriptions, keywords, OpenGraph metadata, and Twitter cards.
Additional SEO strategies employed include structured data markup, internal link optimization, and targeted keyword research. These efforts have shown initial positive impacts on search engine rankings.
Future Enhancements
- > UI design optimization: While AI tools like Cursor provide valuable suggestions, there's significant potential for human-driven improvements based on user feedback and usability studies.
- > Continued SEO refinement: Plans include strategic promotion on platforms like Twitter and Reddit to boost visibility. Ongoing experimentation with keyword optimization and content descriptions is underway to maximize organic reach.
- > Performance optimization: Continuous monitoring and improvement of page load times, server response speeds, and overall application performance.